The terminal is a really powerful tool for developers, and I like the customizability and the amount of optimizations you have access to. But in my opinion, it needs to look good. The default is very unsatisfying to look at. If you have not read Scott Hanselmann’s blog entry on PowerShell customization, I highly recommend checking that out. I don’t use PowerShell very much and prefer using Windows Subsystem For Linux 2 and Ubuntu, but luckily you can do use both with the Windows Terminal.
You can simply set your desired background in the settings pane of Windows Terminal.
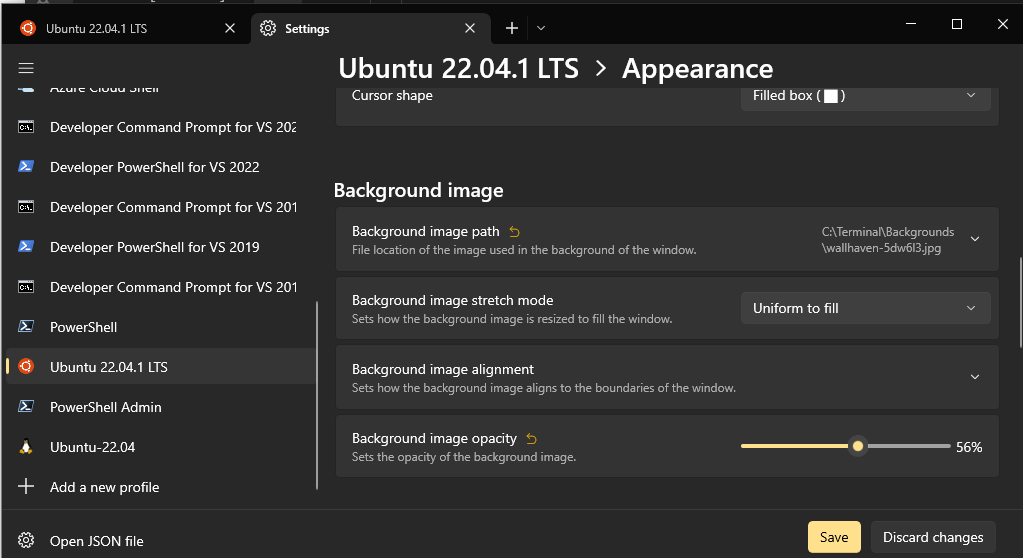
You can also set all of these options in the settings file located at C:\Users\{user}\AppData\Local\Packages\Microsoft.WindowsTerminal_8wekyb3d8bbwe\LocalState\settings.json.
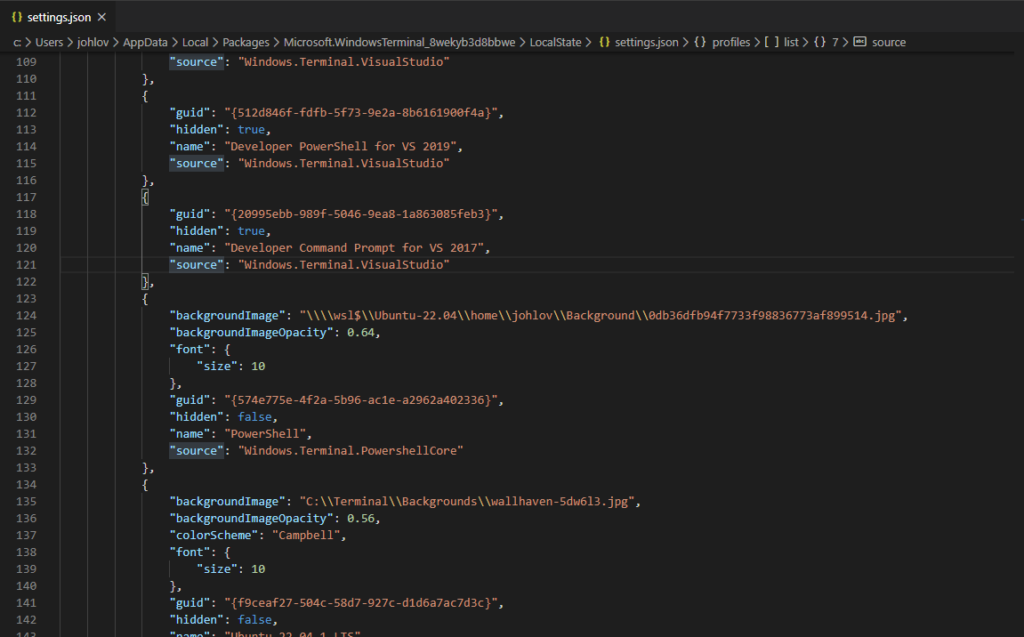
Setting the following values will alter the appearance of the corresponding profile.
{ "backgroundImage": "image.jpg", "backgroundImageOpacity": 0.64 }
Doing this while Windows Terminal is running will update all the terminal instances which use that specific profile. This means that you can change the background programmatically if you want to. You could for instance change it on startup, on a timer, or on a set condition. The sky is the limit! Personally I prefer binding a hotkey for changing the background so I can change the scenery whenever I feel like it.
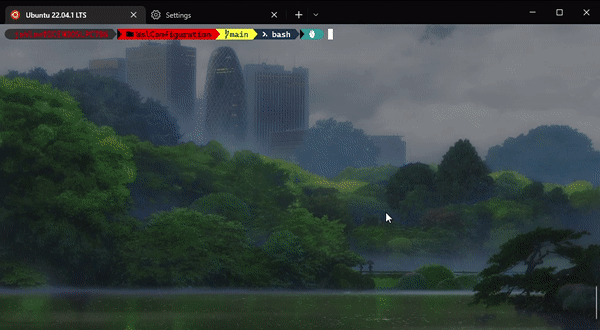
Creating a script for changing background in Windows.
- Get some backgrounds.
- Find the guid of the profile you want to change in settings.json
- Change the “backgroundImage” value to the path of your image.
This in example of doing this in Windows, using WSL2 is a bit tricky as the filepaths are a bit different, so I did not include it. But it shouldn’t be too hard to change it.
SetBackgroundWindows.py
import json import glob import os import re import random ## Get Settings. dirname = os.path.dirname(os.path.realpath(__file__)) f = open(f'{dirname}/settings.json') data = json.load(f) profileGuid=data["ProfileGuid"] terminalSettingsPath = data["TerminalSettingsPath"] backgroundImagePath = data["BackgroundsPath"] # Get JPGs and PNGs from image folder. jpgs = glob.glob(f"{backgroundImagePath}/*.jpg") pngs = glob.glob(f"{backgroundImagePath}/*.png") jpgs.extend(pngs) backgrounds = jpgs f.close() windowsPaths = list(backgrounds) # Update settings. with open(terminalSettingsPath, "r+") as f: data = json.load(f) profiles = data["profiles"]["list"] # Get profile with guid. elem = next(filter(lambda profile: profile["guid"] == profileGuid, profiles), None) random.shuffle(windowsPaths) # Find first non-matching image, don't want duplicates windowsPaths = [value for value in windowsPaths if value != elem["backgroundImage"]] elem["backgroundImage"] = windowsPaths[0] f.seek(0) # <--- should reset file position to the beginning. json.dump(data, f, indent=4) f.truncate()
settings.json
{ "TerminalSettingsPath": "C:\\Users\\Elmer\\AppData\\Local\\Packages\\Microsoft.WindowsTerminal_8wekyb3d8bbwe\\LocalState\\settings.json", "BackgroundsPath": "C:\\Terminal\\Backgrounds", "ProfileGuid": "{61c54bbd-c2c6-5271-96e7-009a87ff44bf}" }
Both files can be found here.
Happy hacking!